Dynamically generate anchor tag in div HTML javascript
Adding any HTML tag within another element
This post will show us how to generate an anchor tag in an existing HTML element. There might be a situation where based on the scenario you want to create a new link anchor tag and assign it within the existing tag like in the ‘<div>’ tag.
Code Snippet
We need to implement a JavaScript function that should be called after the click event. Below are the actual JavaScript lines that create an anchor tag and append it to the existing div tag, during run time.
var existingDivTag = document.getElementById("existingDiv");
var anchorDynTag = document.createElement("a");
anchorDynTag.setAttribute("href","http://YourURLorLink");
anchorDynTag.setAttribute("target","_blank");
anchorDynTag.textContent = "Click here...";
existingDivTag.appendChild(anchorDynTag);
Download this sample and test it yourself – Dynamically generate anchor tags in div.html
How to implement – Steps
Scenario:
After clicking on “Button”, add a link tag(anchor) in an existing div tag.
What do we require?
- Create an “<div>” tag with unique ‘id’ id=”existingDiv”
- Write a JavaScript function that generates a tag during runtime
- Create a button and assign the JavaScript function to the onclick event
- (optional) assign custom CSS
Full HTML code:
<html>
<head>
<style>
.btnCreateLink{
padding : 1rem 2rem 1.0625rem 2rem;
border-width : 0;
background-color : #008CBA;
border-color : #007095;
font-size : 18px !important;
color:white !important;
}
</style>
<script>
function createDynAnchorLink(filename){
var existingDivTag = document.getElementById("existingDiv");
var anchorDynTag = document.createElement("a");
anchorDynTag.setAttribute("href","https://YourDirPath/" + filename + ".pdf");
anchorDynTag.setAttribute("target","_blank");
anchorDynTag.textContent = "Click here to ....whatever....";
existingDivTag.appendChild(anchorDynTag);
existingDivTag.appendChild(document.createElement("br"));
existingDivTag.appendChild(document.createElement("br"));
}
</script>
</head>
<body>
<br><br>
<div id="existingDiv" style="background-color: lightgoldenrodyellow;">
<p>Click on Generate Link button. It will add a new anchor tag in the existing this div tag.</p>
</div>
<br>
<div>
<input type="button" id="cdlbtn" value="Download" onclick="this.disabled=true;createDynAnchorLink('YourFileName')" class="btnCreateLink">
</div>
</body>
</html>
JS Code Explaination
createDynAnchorLink(filename)
During runtime, passing the filename to this function is mandatory and this will be called from the button click event. This is very useful when the ‘path’ location of the file is unique and you just want to replace the file name based on the web or HTML page.
var existingDivTag = document.getElementById(“existingDiv”);
Create div and assign a unique id – existingDiv. The anchor tag will be added after the paragraph tag. In the JS function, assign this div element to a variable called existingDivTag
var anchorDynTag = document.createElement(“a”);
Create a new anchor element and assign it to a variable called anchorDynTag
anchorDynTag.setAttribute(“href”,”https://YourPath/” + filename + “.pdf”);
Set href attribute and give URL link as value for anchor element.
anchorDynTag.setAttribute(“target”,”_blank”);
To open the URL link in a new tab window, add a target attribute.
anchorDynTag.textContent = “Your link text”;
Set text for anchor tag textContent which will be displayed as a text link.
existingDivTag.appendChild(anchorDynTag);
Append the created new anchor tag to the existing div element existingDivTag
existingDivTag.appendChild(document.createElement(“br”));
Additionally, if you want to add a line break after the new anchor element then create br element and append it to the same existing div tag
Screenshot – output
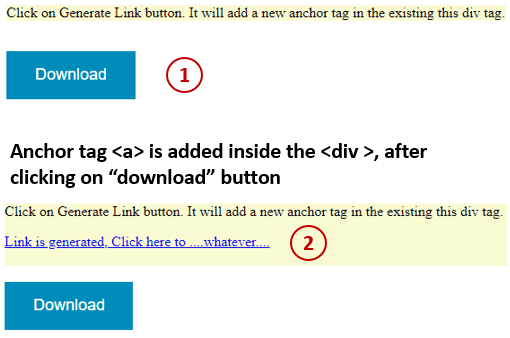
The same code is implemented in the sample file. you can download it and try it yourself.
Thanks 🙂